Sorting Elements Within Filters
This guide explains how to customize the order of filter options in a Findify integration using Liquid. By default, most filter options are sorted dynamically according to the most present values, while there are specific logics for price, and for size.
However, clients may require a custom sorting logic to enhance the user experience. This guide walks through implementing this customization.
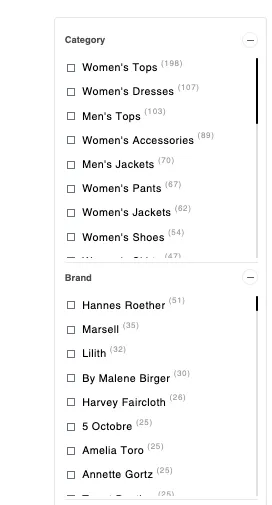
Default Sorting Logic in Findify
Out of the box, Findify applies the following sorting rules:
- Size: Sorted from smallest to largest.
- Price: Groups and order are defined in the Findify dashboard.
- All Other Filters: Sorted by the count of corresponding products. For example, filter options such as sizes or brands with a higher count of products will be displayed first.
Customizing Sorting Logic
To apply custom sorting logic, follow these steps:
1. Access Theme Code
- Navigate to your Shopify admin dashboard.
- Go to Online Store → Themes.
- Choose the theme to edit and click Edit Code.
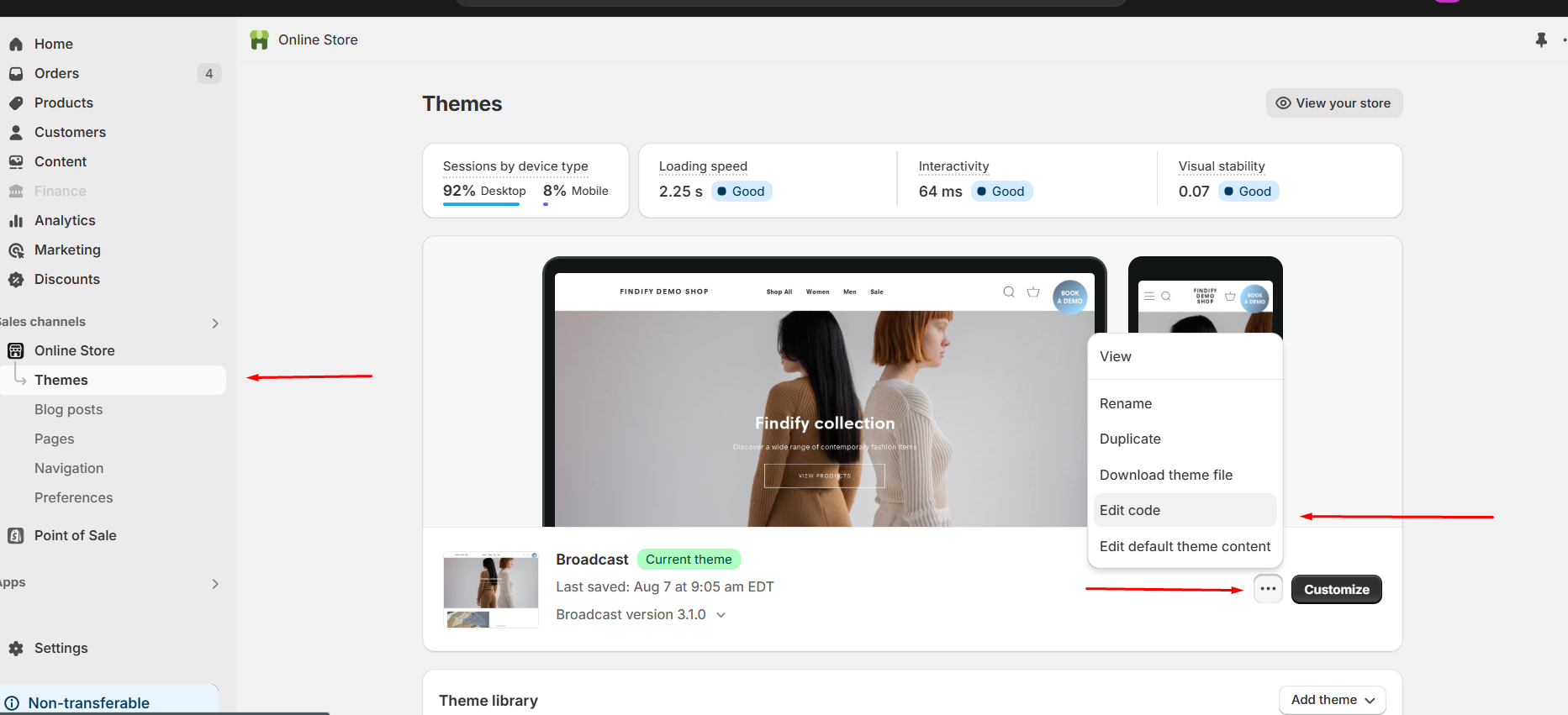
2. Modify Filter Logic
- Locate the file:
assets/findify-filter.js
. - Add the following function at the end of the file to define custom sorting logic:
const sortFilters = (filterName) => {
const filter = document.querySelector(`[data-filter-name="${filterName}"]`)
const body = filter.querySelector('.findify-filters--body-wrapper');
const children = Array.from(body.querySelectorAll('.findify-filters-checkbox-item'));
// Sort filter items based on their 'value' attribute (you can modify this to suit your needs)
children.sort((a, b) => {
const aValue = a.getAttribute('value');
const bValue = b.getAttribute('value');
return aValue - bValue;
});
children.forEach(child => body.appendChild(child));
}
3. Update Filter File
- Open
snippets/findify-filter-text.liquid
. - Add the
data-filter-name
attribute to the.findify-filters—body-wrapper
element. - At the bottom of the file, add the following script to trigger the custom sorting function:
<script>
const filterName = '{{ name }}';
sortFilters(filterName)
</script>
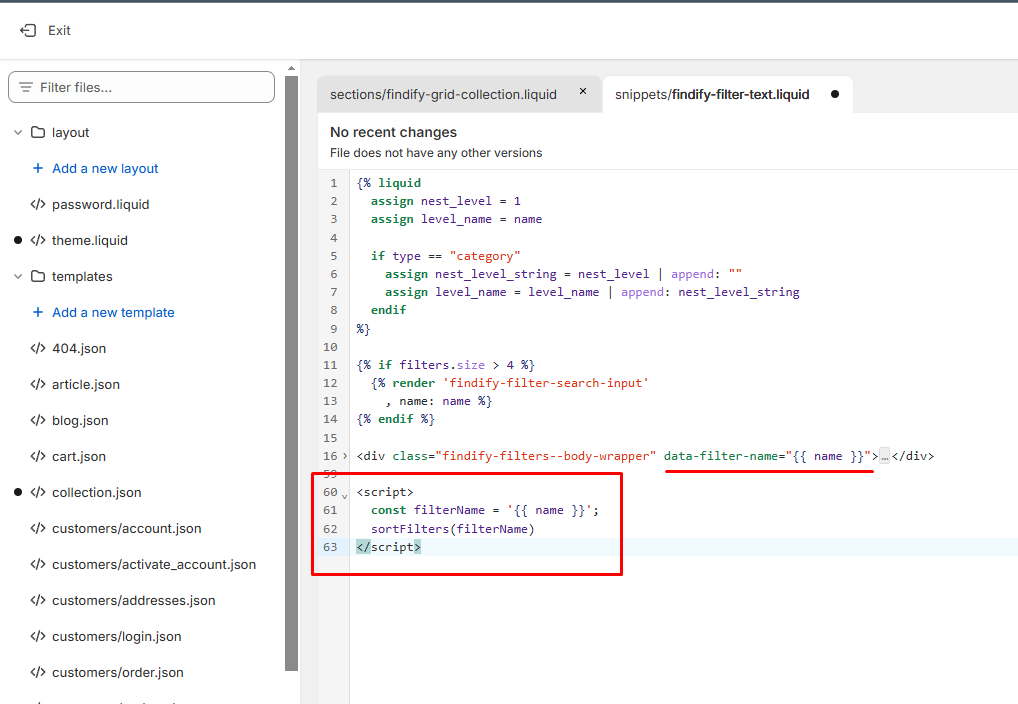
Example: Manual Sorting of Filter Options
To manually specify the order of certain values, define a sorted list of values within the function logic. For instance:
const sortedBrands = ['dell', 'barco', 'eizo', 'amd']; //etc..
const sortFilters = (filterName) => {
const filter = document.querySelector(`[data-filter-name="${filterName}"]`)
const body = filter.querySelector('.findify-filters--body-wrapper');
const children = Array.from(body.querySelectorAll('.findify-filters-checkbox-item'));
switch(filterName.toLowerCase()) {
case 'brand':
children.sort((a, b) => {
const aMake = a.getAttribute('value').toLowerCase();
const bMake = b.getAttribute('value').toLowerCase();
const aIndex = sortedBrands.indexOf(aMake) !== -1 ? sortedBrands.indexOf(aMake) : Infinity;
const bIndex = sortedBrands.indexOf(bMake) !== -1 ? sortedBrands.indexOf(bMake) : Infinity;
return aIndex - bIndex;
});
break;
// more cases if needed
default:
break;
}
children.forEach(child => body.appendChild(child));
}
Before Custom Sorting:
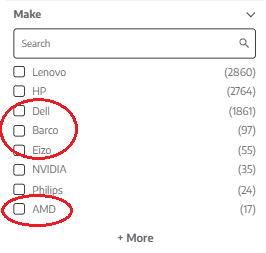
In this example, four values are manually sorted: 'Dell', 'Barco', 'Eizo', and 'AMD'.
After Custom Sorting:
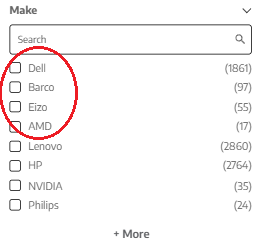
After custom sorting logic is applied, these four values are returned in the defined order.
FAQs
What are the default sorting options in Liquid?
- Size: Smallest to largest.
- Price: Configured in the dashboard.
- Other Filters: Based on the count of corresponding products.
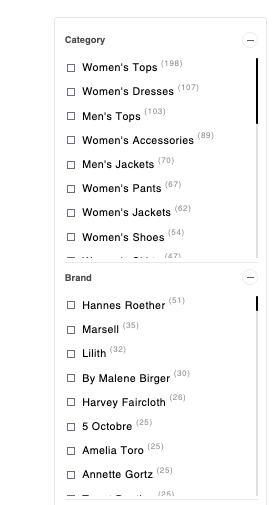
Can sorting logic be customized?
Yes, sorting logic can be configured to arrange options alphabetically, numerically, or based on custom attributes.
For example, for a Findify client that sells computer equipment, where the values are 16GB
, 24GB
, and 1TB
, the sorting logic manipulates these values by converting them into a consistent unit. If the value is in TB, it is multiplied by 1024 to convert it to GB before sorting. This ensures accurate numerical sorting rather than sorting the values directly based on their raw text.
Can I create a manual list of filter values to specify their order?
Yes, by defining a custom array and matching it to filter options in the JavaScript logic.
How do I determine the filter name to be used?
To determine the appropriate filterName
to use in a switch/case
statement, the value of the data-filter-name
attribute can be extracted from DOM.
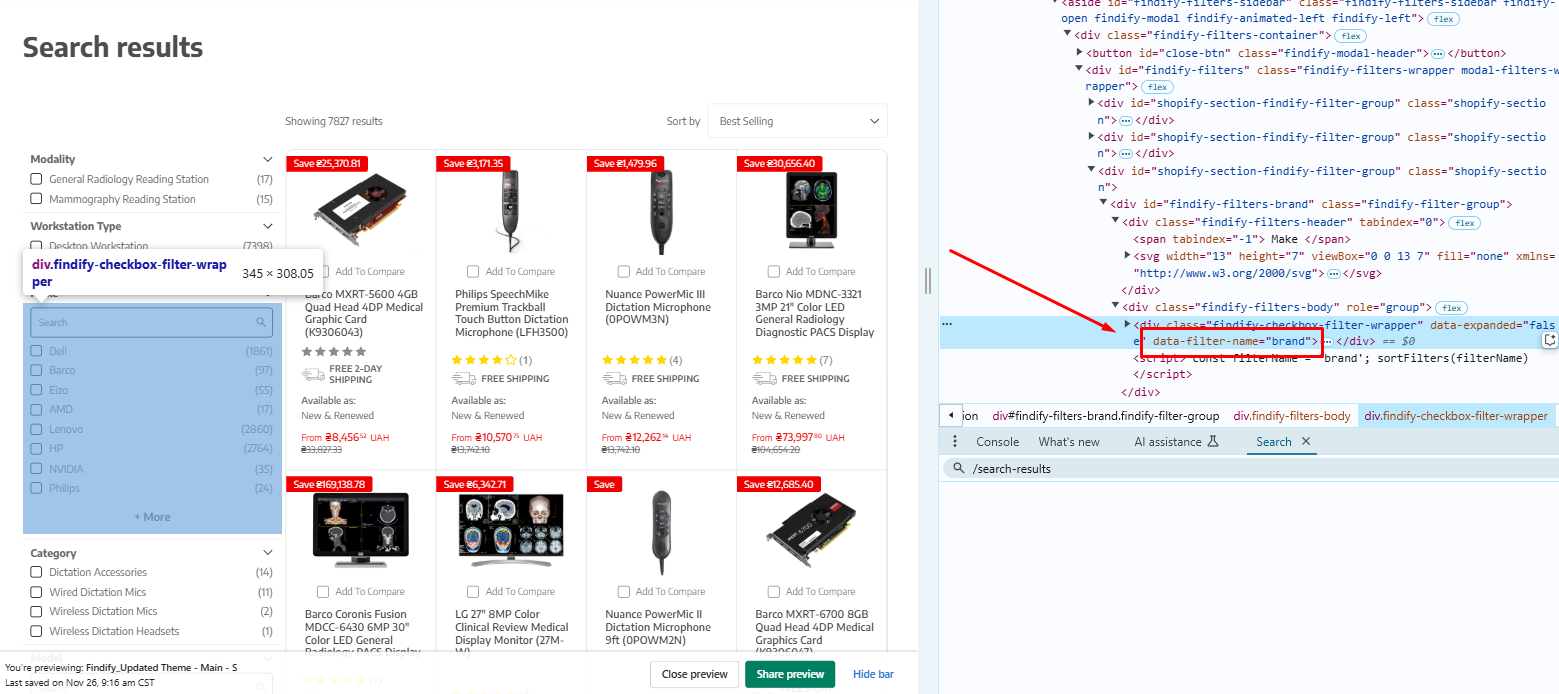
Updated 6 months ago