Alternating amount of products in a recommendation
This example is showcasing how to adjust the recommendations widget for sliders (or grid) to display custom products per row for individual recommendation widgets.
For example, one recommendation widget should display 4 products per row on desktop, another widget should show 3 products per row on desktop.
Firstly, you would need to enable recommendations widgets in Merchant Dashboard.
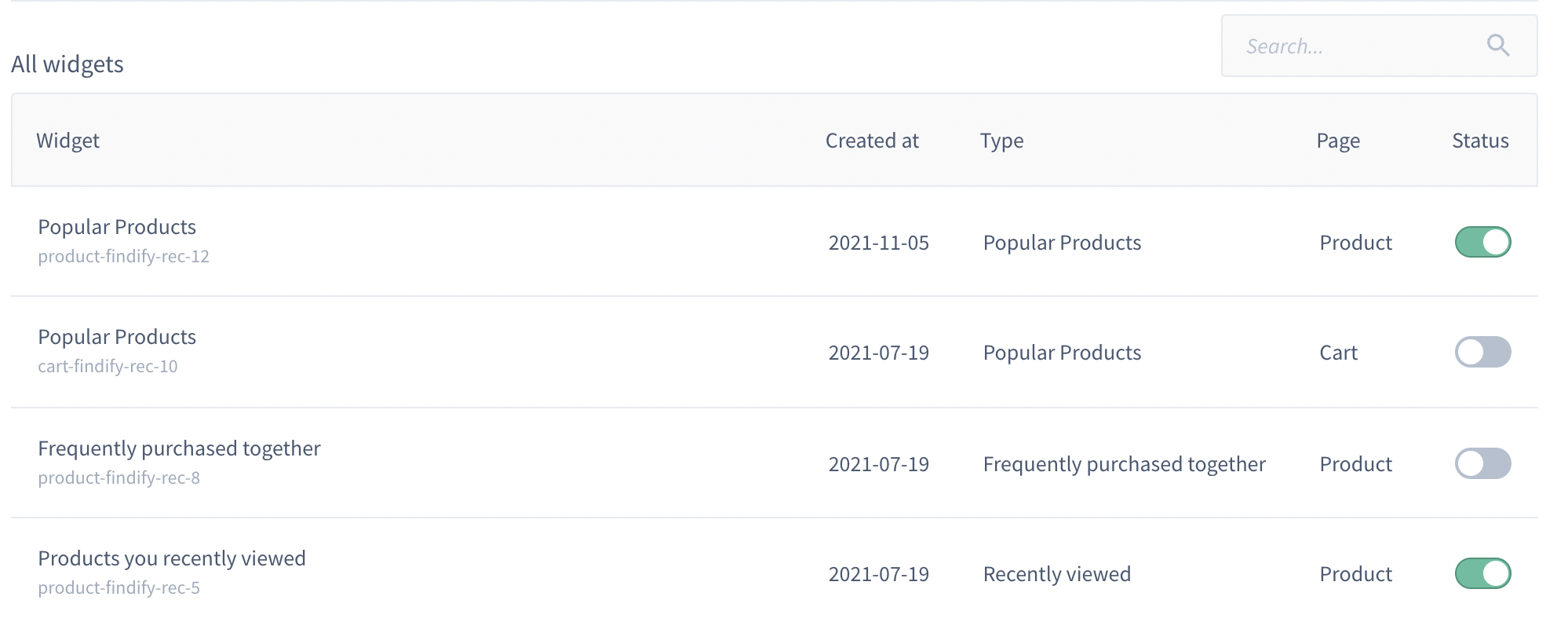
Note
For Slider, you would define how many products you would want per row.
For Grid, you can define the width of the columns, e.g. 12 would be equal to 100% width, 4 to 33.3% width, 3 to 25%, etc.
/**
* @module components/Dropdown
*/
import Swiper from 'components/Swiper';
import ProductCard from 'components/Cards/Product';
import Text from 'components/Text';
import useColumns from 'helpers/useColumns';
import { useItems } from '@findify/react-connect';
import { Immutable } from '@findify/store-configuration';
import useScrollOnChange from 'helpers/useScrollOnChange';
import styles from 'layouts/Recommendation/Slider/styles.css';
const getSliderOptions = (config) => {
/* Push id's of recommendations to array */
const listOfChangedGridRecs = ['product-findify-rec-5'];
const changedRec = listOfChangedGridRecs.find((el) => el === config.get('node').id);
/* Set values for new layout */
let newRecColumns = [
{width: 400, value: 3},
{width: 600, value: 4}
];
/* If there is changedRec then set new state */
const columns = changedRec ? useColumns(newRecColumns) : useColumns(config.getIn(['breakpoints', 'grid']));
return {
spaceBetween: 12,
slidesPerView: 12 / Number(columns),
};
};
export default ({ theme = styles }) => {
const { items, config } = useItems<Immutable.RecommendationConfig>();
const options = getSliderOptions(config);
useScrollOnChange(items);
if (!items?.size) return null;
return (
<>
<Text title component="p" className={theme.title}>
{config.get('title')}
</Text>
<Swiper {...options} slot={config.get('slot')}>
{items
.map((item) => (
<ProductCard
key={item.hashCode()}
item={item}
config={config.get('product')}
/>
))
.toArray()}
</Swiper>
</>
);
};
/**
* @module layouts/Recommendation/Grid
*/
import Grid from 'components/common/Grid';
import Text from 'components/Text';
import { IProduct, MJSConfiguration, ThemedSFCProps } from 'types/index';
import { List } from 'immutable';
import Product from 'components/Cards/Product';
import MapArray from 'components/common/MapArray';
import styles from 'layouts/Recommendation/Grid/styles.css';
import { useItems } from '@findify/react-connect';
import { Immutable } from '@findify/store-configuration';
/** This is a list of props Grid layout for Recommendations accepts */
export interface IGridProps extends ThemedSFCProps {
/** immutable.List of Products to display */
items: List<IProduct>;
/** MJS configuration */
config: MJSConfiguration;
columns: string;
}
export default ({ theme = styles }: IGridProps) => {
const { items, config } = useItems<Immutable.RecommendationConfig>();
if (!items?.size) return null;
/* Push id's of recommendations to array */
const listOfChangedGridRecs = ['product-findify-rec-12'];
/* Set values for new Layout */
let newRecGrid = [
{width: 400, value: 3},
{width: 600, value: 2}
];
/* If there is changedRec then set new state */
const changedRec = listOfChangedGridRecs.find((el) => el === config.get('node').id);
return (
<>
<Text title component="p" className={theme.title}>
{config.get('title')}
</Text>
{/* Check if there is changedRec state */}
<Grid gutter={20} columns={changedRec ? newRecGrid : config.getIn(['breakpoints', 'grid'])}>
{MapArray({
config: config.get('product'),
array: items,
factory: Product,
})}
</Grid>
</>
);
};
Updated over 1 year ago