Load Status Bar
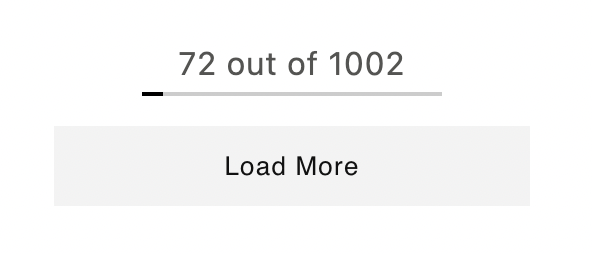
#1. Background
Providing your customer with small yet quite important bits of information regarding what he's browsing can very well be a deal-breaker. Any native way to let him know what's coming down the pike is great.
In this particular case we'll be showing you how to add a Load-status bar, going from 0 to 100% fill depending on how many products on this particular page have been loaded and seen by the customer.
#2. Requirements
- The only requirement for this customisation is to have the Lazy Load enabled for your store.
#3. Time Estimates
Set up in Platform: n/a
Integration: 30 minutes
Styling: 5-10 minutes
#4. Functional Overview
The list of components to be adjusted is as follows:
Components
- LoadBar
- components/search/LazyResults/index.tsx
#5. Integration Steps
Firstly, you'll need the LoadBar function, which is receiving the total amount of products, products per page and percentage of the status bar fill.
Then, we place it within the LazyResults component wherever we want and style it.
NB: you can switch between different components in the below view.
import { useQuery } from '@findify/react-connect';
export const LoadBar = ({productsShown}) => {
const { meta } = useQuery();
const total = meta?.get('total');
const productPerPage = productsShown > total ? total : productsShown;
const percentage = parseInt((productsShown / total) * 100);
return (
<div className="findify-load-bar">
{productPerPage} out of {total}
<div className="findify-bar-wrapper">
<div className="findify-bar-status"></div>
<div className="findify-bar-fill" style={{width: `${percentage}%`}}></div>
</div>
</div>
)
}
{/* ... some code ... */}
const LazyResults = (props) => {
{/* ... some code ... */}
const { getPageProps, current } = usePagination();
const [productsShown, setProductsShown] = useState(0);
useEffect(() => {
const count = config?.getIn(['defaultRequestParams', 'limit']) * current;
return count < productsShown ? '' : setProductsShown(count);
}, [current]);
return (
{/* Put component wherever you want */}
<LoadBar
productsShown={productsShown}
/>
)
}
/* Style width of status bar */
.findify-bar-wrapper {
position: relative;
width: 150px;
}
/* Style background */
.findify-bar-status {
display: block !important;
height: 2px;
width: 100%;
background: #ccc;
}
/* Style fill */
.findify-bar-fill {
display: block !important;
position: absolute;
left: 0;
top: 0;
height: 2px;
background: black;
}
Updated 9 months ago