Mobile Facet using Desktop Filters
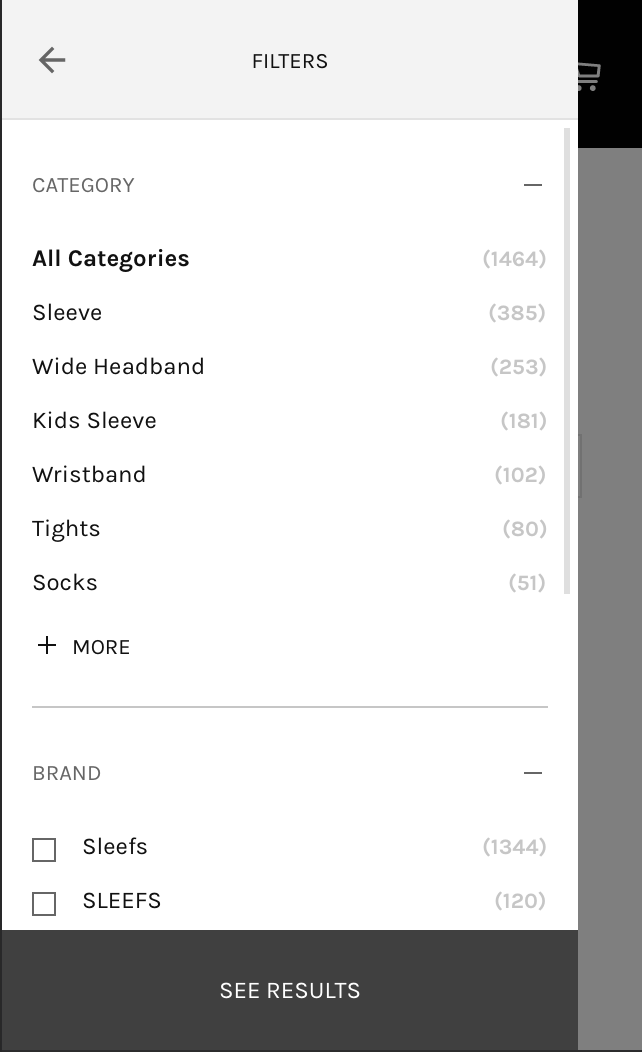
If you don't want to use the default mobile filters but the desktop filter version within the mobile overlay instead, you could do it like this:
// some imports
import Facet from 'components/Facet'; // this is what we use for Desktop filters
// some code
<div className={theme.body}>
// You will find a code snippet like this. Remove <Branch />
/* <Branch
config={config}
theme={theme}
selectFacet={selectFacet}
active={activeFacet}
facets={facets}
condition={!!activeFacet}
right={FacetTitles}
left={FacetContent} /> */
// and instead use this:
<div className='findify-mobilefacet--custom'>
<MapArray
theme={{ root: theme.facet }}
array={facets}
factory={Facet}
config={config}
keyAccessor={i => i.get('name')} />
</div>
// some code
/* adds some basic style to match the desktop layout */
.findify-mobilefacet--custom {
padding: 0 15px;
}
.findify-mobilefacet--custom > div {
border-bottom: 1px solid #e2e2e2;
margin-bottom: 20px;
margin-top: 20px;
padding-bottom: 20px;
}
Adding Breadcrumbs
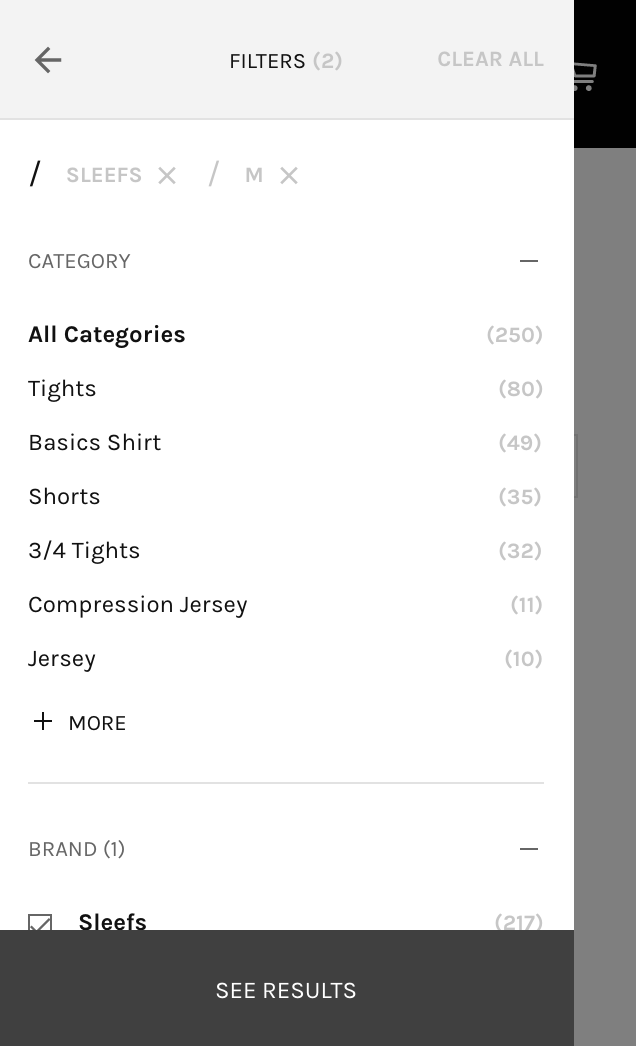
// some imports
import Breadcrumbs from 'components/Breadcrumbs';
// add this where you want the breadcrumbs to appear
<Breadcrumbs theme={{ root: theme.breadcrumbs }} />
.findify-mobilefacet--custom {
margin-top: 15px;
}
.findify-components--breadcrumbs__breadcrumb {
margin-right: 12px;
margin-left: 0;
}
Complete Example
A complete components/search/MobileFacets/view.tsx
could look like this:
/**
* @module components/search/MobileFacets
*/
import React from 'react';
import { withHandlers } from 'recompose';
import Branch from 'components/common/Branch';
import MapArray from 'components/common/MapArray';
import FacetTitles from 'components/search/MobileFacets/Titles';
import Component from 'components/Facet/Component';
import Button from 'components/Button';
import cx from 'classnames';
import Icon from 'components/Icon';
import Text from 'components/Text';
import { ThemedSFCProps, IFacet, MJSConfiguration, MJSValue } from 'types';
import { List } from 'immutable';
// imports
import Facet from 'components/Facet';
import Breadcrumbs from 'components/Breadcrumbs';
/** Props that FacetContent accepts */
export interface IFacetContentProps extends ThemedSFCProps {
/** Currently active facet */
active: IFacet;
/** MJS Configuration */
config: MJSConfiguration;
}
const FacetContent = ({ active, config, theme }: IFacetContentProps) => (
<div className={cx(theme.container, theme[active.get('type') as string])}>
<Component
isExpanded
type={active.get('type')}
facet={active}
config={config}
theme={{
range: theme.range,
expand: theme.expand,
expandedList: theme.expandedList,
}}
isMobile={true}
/>
</div>
);
/** Props that MobileFacets view accepts */
export interface IMobileFacetsProps extends ThemedSFCProps {
/** immutable.List() of Facets */
facets: List<IFacet>;
/** Currently active facet */
activeFacet?: IFacet;
/** Method used to select a facet */
selectFacet: (name?: string) => any
/** Method used to reset facet */
onReset: () => any
/** MJS Configuration */
config: MJSConfiguration;
/** MJS API Request Metadata */
meta: Map<string, MJSValue>;
/** Method used for hiding modal / drawer */
hideModal: (name: string) => any
/** Total filters selected */
total: number;
/** Filters selected for active facet */
filtersSelected: number;
}
export default ({
theme,
facets,
activeFacet,
selectFacet,
onReset,
config,
meta,
hideModal,
total,
filtersSelected,
}: IMobileFacetsProps) =>
<div className={cx(theme.modal, 'mobile')}>
<div className={theme.header}>
<div className={theme.title}>
<Text primary uppercase display-if={!activeFacet}>
{ config.getIn(['facets', 'i18n', 'filters'], 'Filters') }
</Text>
<Text secondary uppercase display-if={!activeFacet && total} className={theme.filterCount}>
({ total })
</Text>
<Text primary uppercase display-if={!!activeFacet}>
{ config.getIn(['facets', 'labels', activeFacet!.get('name')]) }
</Text>
<Text secondary uppercase display-if={!!activeFacet && filtersSelected} className={theme.filterCount}>
({ filtersSelected })
</Text>
</div>
<Button onClick={activeFacet ? selectFacet : hideModal} className={theme.backButton} >
<Icon name='ArrowBack' />
</Button>
<Button
display-if={meta.get('filters') && meta!.get('filters')!.size}
onClick={onReset}>
<Text secondary uppercase>
{ config.getIn(['facets', 'i18n', 'clearAll'], 'Clear All')}
</Text>
</Button>
</div>
<div className={theme.body}>
// div with breadcrumbs and desktop filters
<div className='findify-mobilefacet--custom'>
<Breadcrumbs theme={{ root: theme.breadcrumbs }} />
<MapArray
theme={{ root: theme.facet }}
array={facets}
factory={Facet}
config={config}
keyAccessor={i => i.get('name')} />
</div>
</div>
<Button className={theme.footer} onClick={activeFacet ? selectFacet : hideModal}>
{ config.getIn(['facets', 'i18n', activeFacet ? 'done' : 'showResults'], 'See results')}
</Button>
</div>
/* adds some basic style to match the desktop layout */
.findify-mobilefacet--custom {
padding: 0 15px;
margin-top: 15px;
}
.findify-mobilefacet--custom > div {
border-bottom: 1px solid #e2e2e2;
margin-bottom: 20px;
margin-top: 20px;
padding-bottom: 20px;
}
/* breadcrumbs */
.findify-components--breadcrumbs__breadcrumb {
margin-right: 12px;
margin-left: 0;
}
Updated about 4 years ago