Change Filter Name to Image
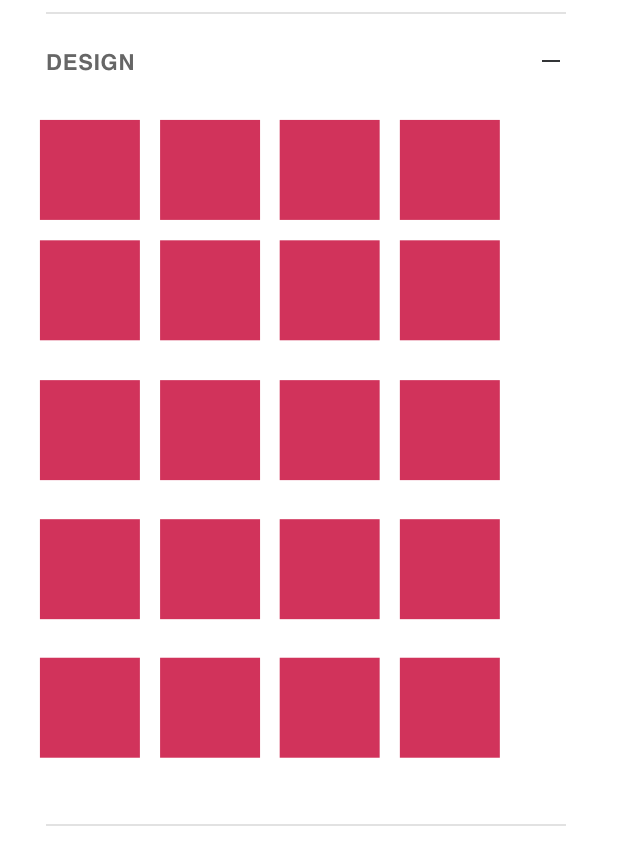
Components:
In this example we are going to change the CheckboxFacet component to show images instead of names in the filters.
First, you need to create an array, where you will add the images for each filter values.
const designImages = {
'<DESIGN>': '<LINK TO IMAGE>',
'<DESIGN 2>': '<LINK TO IMAGE>'
};
Then you need to write a condition, where you will remove the checkbox icons for this specific filter.
(In this example the filter's name is design
which is inside of custom_fields
in the API response.)
<Icon display-if={filterName !== 'custom_fields.design'} name={item.get('selected') ? 'CheckboxFilled' : 'CheckboxEmpty'} />
And then change the text to an image, like this
<Text
primary
lowercase
className={theme.content}
bold={item.get('selected')}>
{ designImages[content({ item })]
? <img src={designImages[content({ item })]} />
: content({ item }) }
</Text>
Final Version
Within our component it could look like this:
/**
* @module components/CheckboxFacet
*/
import React from 'react';
import content from 'components/CheckboxFacet/content';
import Button from 'components/Button';
import Text from 'components/Text';
import Icon from 'components/Icon';
import { IFacetValue, ThemedSFCProps } from 'types';
/** Props that CheckboxFacet Item accepts */
export interface ICheckboxFacetItemProps extends ThemedSFCProps {
/** Single item from facet */
item: IFacetValue;
/** CheckboxFacet Item click handler */
onItemClick?: (evt: Event) => any;
/** Custom inline style */
style: { [x: string]: string | number };
}
const designImages = {
'<DESIGN>': '<LINK TO IMAGE>',
'<DESIGN 2>': '<LINK TO IMAGE>'
};
const Item = ({ item, theme, style, onItemClick,filterName }: ICheckboxFacetItemProps) =>
<Button display-if={ (filterName !== 'custom_fields.design') || (filterName == 'custom_fields.design' && designImages[content({ item })]) } style={style} className={theme.item} onClick={(evt) => {
item.toggle(evt)
onItemClick && onItemClick(evt);
}}>
<Icon display-if={filterName !== 'custom_fields.design'} name={item.get('selected') ? 'CheckboxFilled' : 'CheckboxEmpty'} />
<Text
primary
lowercase
className={theme.content}
bold={item.get('selected')}>
{ designImages[content({ item })]
? <img src={designImages[content({ item })]} />
: content({ item })
}
</Text>
<Text display-if={filterName !== 'custom_fields.design'} secondary uppercase>
({item.get('count')})
</Text>
</Button>
export default Item;
Updated over 4 years ago