Change 'sort by' labels
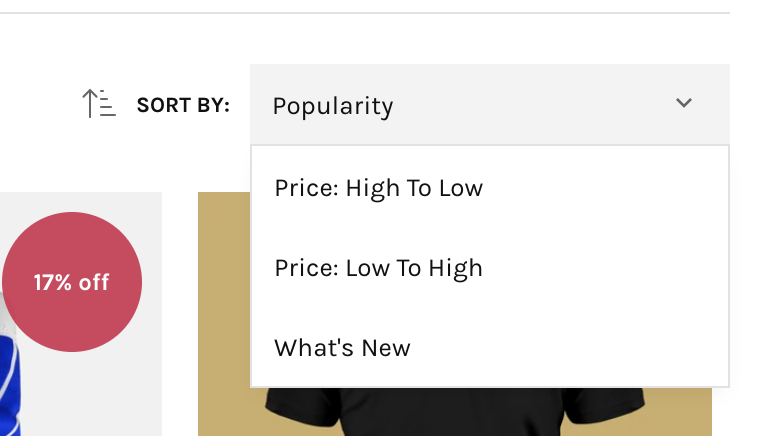
Components:
In order to change the 'sort by' labels, you would need to modify three components mentioned above.
/**
* @module components/Sorting
*/
import React from 'react';
import { connectSort } from '@findify/react-connect';
import { compose, withPropsOnChange, setDisplayName, withHandlers, branch, renderNothing } from 'recompose';
import withTheme from 'helpers/withTheme';
import { is } from 'immutable';
import view from 'components/Sorting/view';
import styles from 'components/Sorting/styles.css';
// same as with the mobileSorting component
const labels = {
"default": "Popularity",
"price|desc": "Price: High to low",
"price|asc": "Price: Low to high",
"created_at|desc": "What's new"
};
export default compose(
setDisplayName('Sorting'),
withTheme(styles),
connectSort,
// remove 'const labels' to use the custom labels we defined above instead of the labels provided by config
withPropsOnChange(['config'], ({ config, selected }) => {
const items = config
.getIn(['sorting', 'options'])
.map(i =>
i.set('label',
labels[[i.get('field'), i.get('order')].filter(i => i).join('|')]
)
);
return { items }
}),
withPropsOnChange(['selected'], ({ items, selected }) => ({
selectedItem: selected && items && items.find(i =>
is(i.get('order'), selected.get('order')) &&
is(i.get('field'), selected.get('field'))
)
})),
withHandlers({
onChangeSort: ({ onChangeSort }) => item =>
item.get('field') === 'default'
? onChangeSort()
: onChangeSort(item.get('field'), item.get('order'))
}),
branch(
({ items }) => !items,
renderNothing
)
)(view);
/**
* @module components/search/MobileSorting
*/
import React from 'react';
import { compose, setDisplayName, withProps, withHandlers } from "recompose";
import { connectSort } from '@findify/react-connect';
import withTheme from 'helpers/withTheme';
import { is } from 'immutable';
import pure from 'helpers/pure';
import view from 'components/search/MobileSorting/view';
import styles from 'components/search/MobileSorting/styles.css';
// add this object to map the default values with whatever values you want. Here is the default values
const labels = {
"default": "Popularity", // if you want to change the popularity, simply change the word 'Popularity' to whatever you want
"price|desc": "Price: High to low",
"price|asc": "Price: Low to high",
"created_at|desc": "What's new"
};
export default compose(
pure,
setDisplayName('MobileSorting'),
withTheme(styles),
connectSort,
// remove 'const labels' to use the custom labels we defined above instead of the labels provided by config
withProps(({ config, meta }) => {
const selected = meta.getIn(['sort', 0]);
const items = config.getIn(['sorting', 'options']).map(i => i
.set('label',
labels[[i.get('field'), i.get('order')].filter(i => i).join('|')]
)
.set('selected',
(!selected && i.get('field') === 'default') ||
!!selected &&
is(i.get('order'), selected.get('order')) &&
is(i.get('field'), selected.get('field'))
)
);
return { items }
}),
withHandlers({
setSorting: ({ items, onChangeSort }) => index =>
onChangeSort(items.getIn([index, 'field']), items.getIn([index, 'order'])),
})
)(view)
/**
* @module components/search/MobileActions
*/
import React from 'react';
import { connectSort, connectQuery } from '@findify/react-connect';
import { compose, withHandlers, withPropsOnChange } from 'recompose';
import withEvents from 'helpers/withEvents';
import withTheme from 'helpers/withTheme';
import view from 'components/search/MobileActions/view';
import styles from 'components/search/MobileActions/styles.css';
// in MobileActions we can adjust the labels to update the labels on the mobile button
const labels = {
"default": "Popularity", // if you want to change the popularity, simply change the word 'Popularity' to whatever you want
"price|desc": "Price: High to low",
"price|asc": "Price: Low to high",
"created_at|desc": "What's new"
};
export default compose(
withTheme(styles),
connectSort,
connectQuery,
withEvents(),
withHandlers({
showFacets: ({ emit }) => () => emit('showMobileFacets'),
showSort: ({ emit }) => () => emit('showMobileSort')
}),
withPropsOnChange(['selected'], ({ selected, config }) => ({
sorting: labels[!!selected
&& [selected.get('field'), selected.get('order')].join('|')
|| 'default'
]
})),
withPropsOnChange(['query'], ({ query }) => query.get('filters') && ({
total: query.get('filters').reduce(
// The workaround to not sum the nested category filters
(acc, filter, key) => acc + (/category[2-9]/.test(key) ? 0 : filter.size)
, 0)
}))
)(view);
If you would like the price labels to say something like "Price $ - $$" or "Price $$ - $", please use the following example as a reference. It is important to use "Price $" + "$ - $" instead of putting two $ signs directly after another, which would cause the store to break.
const labels = {
“default”: “Popularity”,
“price|desc”: “Price $” + “$ - $“,
“price|asc”: “Price $ - $” + “$”,
“created_at|desc”: “What’s new”
};
Updated over 5 years ago