Add Tooltip to Color Facet Values
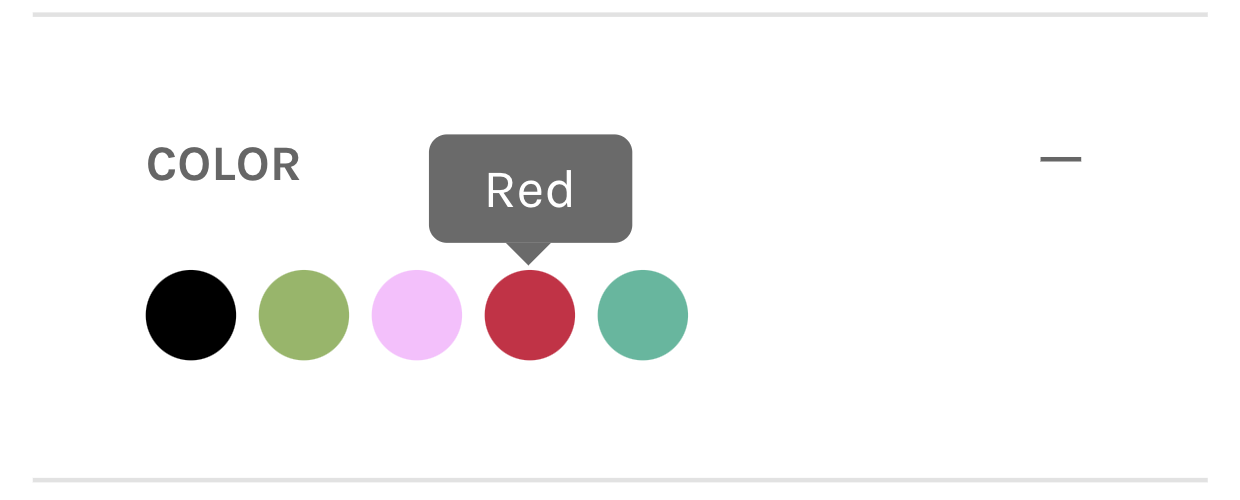
Components
To add a tooltip to color facet values you would require colorFacet/content.tsx
component and a little bit of styling. Here is the example of how to implement the tooltip with some basic styling (of course, it can be changed if needed).
All you have to do is adjust the return statement to include the tooltip as you can see below:
/**
* @module components/ColorFacet
*/
import React from 'react';
// import color from 'tinycolor2';
import { IFacetValue, MJSConfiguration } from 'types';
/**
* Used to retrieve CSS styles for each facet
* @param item Facet value
* @param config MJS configuration to pull mapping from
*/
const getStyles = (item: IFacetValue, config: MJSConfiguration) => {
const value = (item.get('value') as string)!.toLowerCase();
const background = config.getIn(['facets', 'color', 'mapping', value], value);
return {
ball: { background },
// check: { color: color(background).isDark() ? '#fff' : '#333' }
}
};
export default ({ item, config, theme }) => {
const styles = getStyles(item, config);
// change the return statement to include the colors as well as the tooltips
return (
<>
<span style={styles.ball} className={theme.ball}/>
<span className='findify-color-facet-value-tooltip' >{item.get('value')}</span>
</>
)
}
.findify-color-facet-value-tooltip {
position: absolute;
bottom: auto;
left: 50%;
top: -45px;
transform: translate(-50%,15px);
border-radius: 4px;
color: #fff;
font-size: 12px;
padding: .5em 1em;
white-space: nowrap;
margin-bottom: 11px;
background-color: hsla(0,0%,7%,.9);
text-transform: capitalize;
transition: transform, opacity .3s;
opacity: 0;
}
.findify-color-facet-value-tooltip:after {
content: " ";
position: absolute;
border-left: 5px solid transparent;
border-right: 5px solid transparent;
border-bottom: 5px solid hsla(0,0%,7%,.9);
top: 24px;
left: 50%;
margin-left: -5px;
transform: rotate(180deg);
}
.findify-components--color-facet__item:hover .findify-color-facet-value-tooltip {
opacity: .7;
}
Updated over 5 years ago