Quick Add & Quick View
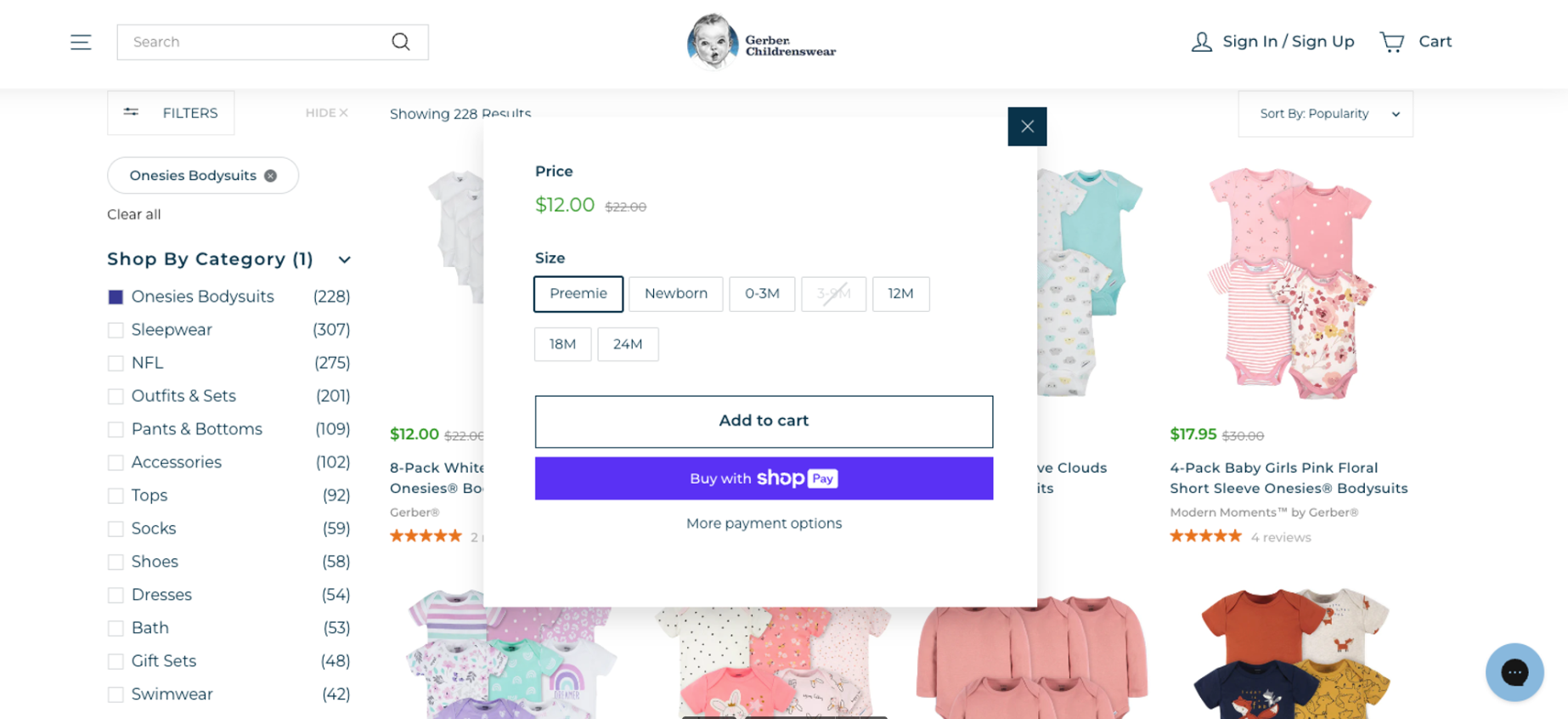
A Quick Add / Quick View feature enhances user experience by allowing seamless access to product details and quick addition to the cart without navigating away from the current page. This functionality can be integrated into Shopify stores using Findify's app, providing flexibility for both themes with existing modals and those without.
Passing Liquid Variables
To implement the Quick Add / Quick View feature, Liquid variables extracted from findify-product-card.liquid
can be transmitted to a JavaScript function. This allows for dynamic population of product information within the modal window.
Example 1: Basic Implementation
First, create a button with onclick function.
Note: You can get values from Shopify itself, using
product.
prefix.
<button
onclick="showQuickAddModal({
brand: '{{ brand }}',
title: '{{ product.title }}',
...other props
})"
>
Quick view
</button>
Example 2: Handling Complex Data
In some cases, values cannot be directly passed, leading to an Unexpected token
error. To prevent this issue, it's necessary to convert the values before passing them. For instance, images should be converted into an array, and product description HTML should be encoded as a URL. Specifically regarding images, only the image URLs will be extracted for passing.
{% assign mediaSrcArray = product.media | json %}
{% assign description = product.description | url_encode %}
<button
onclick="showQuickAddModal({
brand: '{{ brand }}',
title: '{{ product.title }}',
images: [{% for image in product.media %}'{{ image | image_url }}',{% endfor %}],
description: '{{ description }}'
})"
>
Quick view
</button>
Creating Modal
- Add a new section named
findify-quick-add.liquid
. - Incorporate this section into
theme.liquid
using{% section 'findify-quick-add' %}
. - Generate a modal template within the section file, incorporating Liquid variables into the markup.
<div class="modal-wrapper">
<div id="modal-product-brand"></div>
<div id="modal-product-description"></div>
<!-- Other variables -->
</div>
<script>
function showQuickAddModal({
id,
images,
brand,
title,
description
}) {
const productBrandDOM = document.getElementById('modal-product-brand');
const productDescriptionDOM = document.getElementById('modal-product-description');
// Other variable declarations
productBrandDOM.innerText = brand;
productDescriptionDOM.innerHTML = decodeURIComponent(description.replace(/\\+/g, ' '))
}
</script>
Passing Product Variants
Product variants can also be passed to the JavaScript function, allowing for dynamic display of variant options within the modal.
Note: Pass them as a string by default.
Example:
<button
onclick="showQuickAddModal({
variants: '{{ variants }}',
...other variables
})"
>
Quick view
</button>
Handling Variant Data
The string representation of variants is parsed into an array of objects using regular expressions to extract relevant information.
function showQuickAddModal({
variants
}) {
/* input: availability=true~color=White~id=39730240159949~price=248~quantity=2~size=X-Small;availability=true... */
const variantsArray = parseVariants(variants);
/* output: [ { availability: true, id: 1, price: ... }, {}, ...] */
}
function parseVariants(variants) {
const objectsArray = variants.split(";").filter(Boolean);
const extractedArray = objectsArray.map(objectString => {
const idMatch = objectString.match(/id=(\\d+)/);
const sizeMatch = objectString.match(/size=([^\\|]+)/);
const priceMatch = objectString.match(/price=(\\d+)/);
const quantityMatch = objectString.match(/quantity=(\\d+)/);
const availabilityMatch = objectString.match(/availability=true/);
/* other regular expressions */
return {
id: idMatch[1],
size: sizeMatch[1],
availability: !!availabilityMatch,
quantity: quantityMatch ? parseInt(quantityMatch[1]) : 0,
price: priceMatch[1],
};
});
return extractedArray;
}
For further assistance or inquiries, feel free to contact us on [email protected].
Updated 18 days ago